I am trying to make a song app in SwiftUI with Firebase so far everything’s going good. I connected the albums to Firebase and it works but the images are the same for each songCell. Here’s what I mean.
In that screen shot each songRow has the same image but I want them to be obviously different images.
Here is the code for it
class OurData : ObservableObject {
@Published public var albums = [Album]()
func loadAlbums (){
Firestore.firestore().collection("albums").getDocuments { snapshot, error in
if error == nil {
for document in snapshot!.documents {
let name = document.data()["name"] as? String ?? "error"
let image = document.data()["image"] as? String ?? "1"
print(image)
print(document.data())
let songs = document.data()["songs"] as? [String : [String : Any]]
var songsArray = [Song]()
if let songs = songs {
for song in songs {
let songName = song.value["name"] as? String ?? "error"
let songTime = song.value["time"] as? String ?? "error"
let songImage = song.value["image"] as? String ?? "3"
songsArray.append(Song(name: songName, time: songTime, songImage: songImage))
}
}
self.albums.append(Album(name: name, image: image, songs: songsArray))
}
} else {
print(error)
}
}
}
}
And here is an image of the Firebase collection
I have 6 images for 6 different songs, 3 in each album here is an image
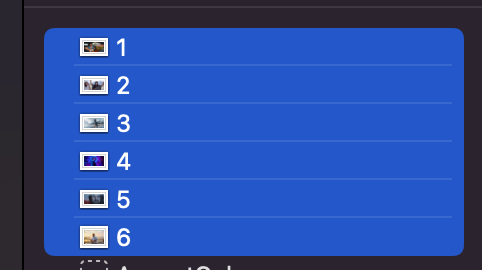
How can I fix this?
if you are using Firebase then ideally I would imagine that you would want to store the images in Firebase Storage for each song and then store a reference to that image data with your song collection. Does that sound like a reasonable assumption?
I tried to do that but it’s not working below is a screenshot of the collection. And I have stored the images also.
@Air_Walks
Where are the mp3 files stored and what code do you have to store the images in Firebase?
The mp3 files are stored here same with the images
I don’t have any code in my Xcode workspace for the images though since I couldn’t find a way to code it.
@Air_Walks
OK so you have uploaded the song image and the song mp3 file to Storage for each song.
What you need to do is write code to save the image and save the associated mp3 file to Storage and then in each case get a reference to each of those stored files and save the url as a string in your albums collection for that song.
The code to do that is quite verbose but that’s the way Firebase rolls.
The way I would approach that is to create a function to save the image and a function to save the mp3 file and in each case use a completion handler to return the url string of each saved file which you can then save to the album collection. For example you might store the image path as an imageUrl
and the mp3 path a songUrl
.
Does that make sense?
I have already written the code for the mp3 file in the storage here it is
func playSong() {
let storage = Storage.storage().reference(forURL: self.song.file)
storage.downloadURL { (url, error) in
if error != nil {
print(error)
} else {
do {
try AVAudioSession.sharedInstance().setCategory(AVAudioSession.Category.playback)
} catch {
// report for an error
}
print(url?.absoluteString)
player = AVPlayer(playerItem: AVPlayerItem(url: url!))
player.play()
}
}
}
func playPause() {
self.isPlaying.toggle()
if isPlaying == false {
player.pause()
} else {
player.play()
}
}
func next() {
if let currentIndex = album.songs.firstIndex(of: song) {
if currentIndex == album.songs.count - 1 {
} else {
player.pause()
song = album.songs[currentIndex + 1]
self.playSong()
}
}
}
func previous() {
if let currentIndex = album.songs.firstIndex(of: song) {
if currentIndex == 0 {
} else {
player.pause()
song = album.songs[currentIndex - 1]
self.playSong()
}
}
}
}
I also tried to save the image but it didn’t change anything in the simulator here is the code
func saveImage() {
let storage = Storage.storage().reference(forURL: self.song.songImage)
storage.downloadURL { (url, error) in
if error != nil {
print(error)
}
}
}
Update*
I successfully fixed the issue on my own by testing and stuff. Thanks for the help.